Foreword
1. Strings and Arrays
- What is string immutability?
- How does substring() work?
- Why string is immutable?
- Create Java string Using ” ” or constructor?
- Is string passed by reference?
- length vs. length()
- How to check if an array contains a value efficiently?
- What is varargs?
- What exactly is null?
- Comparator vs. Comparable
- The contract between hashCode() and equals()
- Java passes object by reference or by value?
- Iteration vs. recursion
- Overloading vs. overriding
- What is instance initializer?
- Fields can not be overridden?
- Inheritance vs. composition
- How to use Java Enum?
- How many types of inner classes?
- What is inner interface?
- Constructors of sub and super classes?
- 4 access levels
- When to use private constructors?
- Class hierarchy of Collection and Map
- ArrayList vs. LinkedList vs. Vector
- HashSet vs. TreeSet vs. LinkedHashSet
- HashMap vs. TreeMap vs. HashTable vs. LinkedHashMap
- A TreeSet example
- Efficient counter
- Frequently used methods of HashMap
- Deep understanding of Arrays.sort(T[], Comparator < ? super T > c)
- How do developers sort?
- java.util.ConcurrentModificationException
- Why do we need generic types?
- Why do we need generic methods?
- Generic Types vs. Generic Methods
- What is type erasure?
- Set vs. Set<?>
- What's the best way of converting Array to ArrayList?
6. Concurrency
- Monitors – the key idea of Java synchronization
- How to make a method thread-safe?
- join()
- notify() and wait()
- Create thread by overriding
- Read file line by line
- Write file line by line
- FileOutputStream vs. FileWriter
- Should .close() be put in finally block or not?
- Java serialization
- How to use properties file?
- JVM run-time data areas
- How does Java handle aliasing?
- What does an array look like in memory?
- What is memory leak?
- What can be learned from "Hello World"?
- Whan and how a class is loaded and initialized?
- Static type checking
- Generate code for overloaded and overridden methods?
- Top 10 mistakes Java developers make
- Top 10 methods for Java arrays
- Top 10 questions of Java strings
- Top 10 questions for Java regular expression
- Top 10 questions about Java exceptions
- Top 10 questions about Java collections
- Top 9 questions about Java maps
- The most widely used Java libraries
- Top 10 websites for advanced level Java developers
- Top 10 books for advanced level Java developers
- Top 100 High-quality Java blogs
- Top 10 algorithms for coding interview
- Top 8 diagrams for understanding Java
- Top 100 Java Classes
- Reflection tutorial
- What is framework?
- Why do we need Java web frameworks like Struts 2?
- What is Servlet Container? What is Tomcat?
- What is aspect-oriented Programming?
- Library vs. framework?
- Spring
- Open source projects using Spring framework
- Design patterns in stories
- Struts 2
- How CVS works?
- Why do we need software testing?
- Convert java jar file to exe
- Guava
- Log4j
- JSoup
- Swing
- Compile and Run Java in Command Line with External Jars
- How to build your own library?
- How to get double?
- Java and computer science courses
- Java vs. Python: Basic Syntax, Date Types
- How to write a crawler?
- 8 things programmers can do at weekends
- Declaration, initialization and scoping
- Date formatting(add more)
- Path of package and class
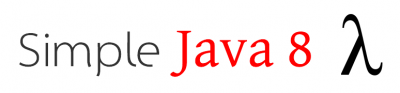
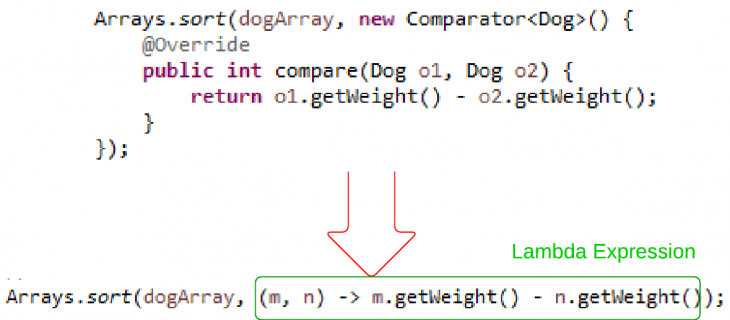
Simple Java 8 Lambdas is a collection of code examples which can help developers quickly start programming lambda expressions in Java 8. Each post illustrates one feature/issue which normally can be completed in 5 minutes. The goal is to make the lambda expression learning simple.
All code used can be downloaded from Github.
1. Lambdas
2. Stream
- What is Stream?
- How Functional Interface Works?
- Create a Stream
- Transform Stream
- Reduce Stream
- Optional Type
- Primitive Type Streams
- How to write a counter in one line of code?
- Enhanced For-loop vs. forEach()
- How to convert a Stream to an array?
- How to concat 2 Streams?
- How to retrieve a List from a Stream?
- Why Stream.max(Integer::max) compiles?
- Return a Stream vs. Return a Collection
1. Difference between HashMap and ConcurrentHashMap Collection ?
HashMap class extends AbstractMap, whereas Hashtable extends the obsolete class Dictionary.
and the popular class Properties extends Hashtable class neither HashMap nor ConcurrentHashMap
Initial capacity of hashtable is 11 while it is 16 in case of hashmap..
hashtable not allowed any null keys or any null value where as hashmap can have one null key and any more null values..
You can use LinkedHashMap with HashMap so you can have predictable iteration which is inseration order by default.
Another difference is that iterator in the HashMap is fail-safe while the enumerator for the Hashtable isn't
HashMap is faster than HashTable -- HashMap is unsynchronized while hash table is synchronized
First and most significant different between Hashtable and HashMap is that, HashMap is not thread-safe while Hashtable is a thread-safe collection.
Second important difference between Hashtable and HashMap is performance, since HashMap is not synchronized it perform better than Hashtable.
Third difference on Hashtable vs HashMap is that Hashtable is obsolete class and you should be using ConcurrentHashMap in place of Hashtable in Java.
2. Difference between HashMap and ConcurrentHashMap in Java ?
first significant difference between HashMap and ConcurrentHashMap is that later is thread-safe and can be used in concurrent environment without external synchronization
You can make HashMap synchronized by wrapping it on Collections.synchornizedMap(HashMap) which will return a collection which is almost equivalent to Hashtable, where every modification operation on Map is locked on Map object while in case of ConcurrentHashMap, thread-safety is achieved by dividing whole Map into different partition based upon Concurrency level and only locking particular portion instead of locking whole Map.
ConcurrentHashMap is more scalable and performs better than Synchronized HashMap in multi-threaded environment while in Single threaded environment both HashMap and ConcurrentHashMap gives comparable performance, where HashMap only slightly better.
In Summary Main difference between ConcurrentHashMap and HashMap in Java Collection turns out to be thread-safety, Scalability and Synchronization.
ConcurrentHashMap is better choice than synchronized HashMap if you are using them as cache, which is most popular use case of a Map in Java application. ConcurrentHashMap is more scalable and outperform when number of reader threads outnumber number of writer threads
3. How to traverse iterate or loop ArrayList in Java ?
Iterating, traversing or Looping ArrayList in Java means accessing every object stored in ArrayList and performing some operations like printing them. There are many ways to iterate, traverse or Loop ArrayList in Java e.g. advanced for loop, traditional for loop with size(), By using Iterator and ListIterator along with while loop etc. All the method of Looping List in Java also applicable to ArrayList because ArrayList is an essentially List. In next section we will see code example of Looping ArrayList in Java.
There are multiple ways to traverse, iterate or loop ArrayList in Java, let’s see some concrete code example to know exactly How to loop ArrayList in Java. I prefer advanced for loop added in Java 1.5 along with Autoboxing, Java Enum, Generics, Varargs and static import, also known as foreach loop if I have to just iterate over Array List in Java. If I have to remove elements while iterating than using Iterator or ListIterator is best solution.
import java.util.ArrayList;
import java.util.Iterator;
/**
* Java program which shows How to loop over ArrayList in Java using advanced for loop,
* traditional for loop and How to iterate ArrayList using Iterator in Java
* advantage of using Iterator for traversing ArrayList is that you can remove
* elements from Iterator while iterating.
* @author
*/
public class ArrayListLoopExample {
public static void main(String args[]) {
//Creating ArrayList to demonstrate How to loop and iterate over ArrayList
ArrayList<String> games = new ArrayList<String>(10);
games.add("Cricket");
games.add("Soccer");
games.add("Hockey");
games.add("Chess");
System.out.println("original Size of ArrayList : " + games.size());
//Looping over ArrayList in Java using advanced for loop
System.out.println("Looping over ArrayList in Java using advanced for loop");
for(String game: games){
//print each element from ArrayList
System.out.println(game);
}
//You can also Loop over ArrayList using traditional for loop
System.out.println("Looping ArrayList in Java using simple for loop");
for(int i =0; i<games.size(); i++){
String game = games.get(i);
}
//Iterating over ArrayList in Java
Iterator<String> itr = games.iterator();
System.out.println("Iterating over ArrayList in Java using Iterator");
while(itr.hasNext()){
System.out.println("removing " + itr.next() + " from ArrayList in Java");
itr.remove();
}
System.out.println("final Size of ArrayList : " + games.size());
}
}
Output:
original Size of ArrayList : 4
Looping over ArrayList in Java using advanced for loop
Cricket
Soccer
Hockey
Chess
Looping ArrayList in Java using simple for loop
Iterating over ArrayList in Java using Iterator
removing Cricket from ArrayList in Java
removing Soccer from ArrayList in Java
removing Hockey from ArrayList in Java
removing Chess from ArrayList in Java
final Size of ArrayList : 0
import java.util.Iterator;
/**
* Java program which shows How to loop over ArrayList in Java using advanced for loop,
* traditional for loop and How to iterate ArrayList using Iterator in Java
* advantage of using Iterator for traversing ArrayList is that you can remove
* elements from Iterator while iterating.
* @author
*/
public class ArrayListLoopExample {
public static void main(String args[]) {
//Creating ArrayList to demonstrate How to loop and iterate over ArrayList
ArrayList<String> games = new ArrayList<String>(10);
games.add("Cricket");
games.add("Soccer");
games.add("Hockey");
games.add("Chess");
System.out.println("original Size of ArrayList : " + games.size());
//Looping over ArrayList in Java using advanced for loop
System.out.println("Looping over ArrayList in Java using advanced for loop");
for(String game: games){
//print each element from ArrayList
System.out.println(game);
}
//You can also Loop over ArrayList using traditional for loop
System.out.println("Looping ArrayList in Java using simple for loop");
for(int i =0; i<games.size(); i++){
String game = games.get(i);
}
//Iterating over ArrayList in Java
Iterator<String> itr = games.iterator();
System.out.println("Iterating over ArrayList in Java using Iterator");
while(itr.hasNext()){
System.out.println("removing " + itr.next() + " from ArrayList in Java");
itr.remove();
}
System.out.println("final Size of ArrayList : " + games.size());
}
}
Output:
original Size of ArrayList : 4
Looping over ArrayList in Java using advanced for loop
Cricket
Soccer
Hockey
Chess
Looping ArrayList in Java using simple for loop
Iterating over ArrayList in Java using Iterator
removing Cricket from ArrayList in Java
removing Soccer from ArrayList in Java
removing Hockey from ArrayList in Java
removing Chess from ArrayList in Java
final Size of ArrayList : 0
That's all on How to iterate, traverse or loop ArrayList in Java. In summary use advance for loop to loop over ArrayList in Java, its short, clean and fast but if you need to remove elements while looping use Iterator to avoid ConcurrentModificationException.
Best way to iterate through ArrayList is via ListIterator because it allows you to add element between current and next element using add() method, replace current element using set() method and remove current element using remove() method. It is also safe, because it can throw ConcurrentModificationException if ArrayList is modified after iterator begins.
Best way to iterate through ArrayList is via ListIterator because it allows you to add element between current and next element using add() method, replace current element using set() method and remove current element using remove() method. It is also safe, because it can throw ConcurrentModificationException if ArrayList is modified after iterator begins.
4. Difference between TreeMap and TreeSet in Java ?
Main Difference between TreeMap and TreeSet is that TreeMap is an implementation of Map interface while TreeSet is an implementation of Set interface.
Key point to remember about TreeMap and TreeSet is that they use compareTo() or compare() method to compare object, So if uses puts a String object in TreeSet of Integers, add() method will throw ClassCastException at runtime prior to Java 5, with Java 5 you can use Generics to avoid this happening by declaring TreeMap and TreeSet with parametrized version.
http://www.amazon.com/dp/0596527756/?tag=javamysqlanta-20
1) Major difference between TreeSet and TreeMap is that TreeSet implements Set interface while TreeMap implements Map interface, which is not a Collection.
2) Second difference between TreeMap and TreeSet is the way they store objects. TreeSet stores only one object while TreeMap uses two objects called key and Value. Objects in TreeSet are sorted while keys in TreeMap remain in sorted Order.
3) Third difference between TreeSet and TreeMap is that, former implements NavigableSet while later implements NavigableMap in Java.
4) Fourth difference is that duplicate objects are not allowed in TreeSet but duplicates values are allowed in TreeMap.
5. Similarities between TreeMap and TreeSet in Java ?
1) Both TreeMap and TreeSet are sorted data structure, which means they keep there element in predefined Sorted order. Sorting order can be natural sorting order defined by Comparable interface or custom sorting Order defined by Comparator interface. Both TreeMap and TreeSet has overloaded constructor which accept a Comparator, if provided all elements inside TreeSet or TreeMap will be compared and Sorted using this Comparator.
2) Both TreeSet and TreeMap implements base interfaces e.g. TreeSet implements Collection and Set interface so that they can be passed to method where a Collection is expected and TreeMap implements java.util.Map interface, which means you can pass it when a Map is expected.
3) TreeSet is practically implemented using TreeMap instance, similar to HashSet which is internally backed by HashMap instance. See my post Internal Implementation of HashSet to learn more.
3) TreeSet is practically implemented using TreeMap instance, similar to HashSet which is internally backed by HashMap instance. See my post Internal Implementation of HashSet to learn more.
4) Both TreeMap and TreeSet are non synchronized Collection, hence can not be shared between multiple threads. You can make both TreeSet and TreeMap synchronized by wrapping them into Synchronized collection by calling Collections.synchroinzedMap() method.
5) Iterator returned by TreeMap and TreeSet are fail-fast, means they will throw ConcurrentModificationException when TreeMap or TreeSet is modified structurally once Iterator is created. this fail-fast behavior is not guaranteed but works in best effort.
6) Both TreeMap and TreeSet are slower than there Hash counter part like HashSet and HashMap and instead of providing constant time performance for add, remove and get operation they provide performance in O(log(n)) order.
TreeMap implemets the sorted Interface,while Hash map not implements the sorted interface.
Treemap allows 'Homogenious' values as keys,where hash map not.
Treemap allows 'Homogenious' values as keys,where hash map not.
7. How to sort ArrayList ascending descending order ?
Sorting ArrayList in Java is not difficult, by using Collections.sort() method you can sort ArrayList in ascending and descending order in Java. Collections.sort() method optionally accept a Comparator and if provided it uses Comparator's compare method to compare Objects stored in Collection to compare with each other, in case of no explicit Comparator, Comparable interface's compareTo() method is used to compare objects from each other. If object's stored in ArrayList doesn't implements Comparable than they can not be sorted using Collections.sort() method in Java.
Here is a complete code example of How to sort ArrayList in Java, In this Sorting we have used Comparable method of String for sorting String on there natural order, You can also use Comparator in place of Comparable to sort String on any other order than natural ordering e.g. in reverse order by using Collections.reverseOrder() or in case insensitive order by using String.CASE_INSENSITIVE_COMPARATOR.
import java.util.ArrayList;
import java.util.Collections;
/**
*
* Java program to demonstrate How to sort ArrayList in Java in both ascending
* and descending order by using core Java libraries.
*
* @author Javin
*/
public class CollectionTest {
public static void main(String args[]) {
//Creating and populating ArrayList in Java for Sorting
ArrayList<String> unsortedList = new ArrayList<String>();
unsortedList.add("Java");
unsortedList.add("C++");
unsortedList.add("J2EE");
System.err.println("unsorted ArrayList in Java : " + unsortedList);
//Sorting ArrayList in ascending Order in Java
Collections.sort(unsortedList);
System.out.println("Sorted ArrayList in Java - Ascending order : " + unsortedList);
//Sorting ArrayList in descending order in Java
Collections.sort(unsortedList, Collections.reverseOrder());
System.err.println("Sorted ArrayList in Java - Descending order : " + unsortedList);
}
}
Output:
unsorted ArrayList in Java : [Java, C++, J2EE]
Sorted ArrayList in Java - Ascending order : [C++, J2EE, Java]
Sorted ArrayList in Java - Descending order : [Java, J2EE, C++]
import java.util.Collections;
/**
*
* Java program to demonstrate How to sort ArrayList in Java in both ascending
* and descending order by using core Java libraries.
*
* @author Javin
*/
public class CollectionTest {
public static void main(String args[]) {
//Creating and populating ArrayList in Java for Sorting
ArrayList<String> unsortedList = new ArrayList<String>();
unsortedList.add("Java");
unsortedList.add("C++");
unsortedList.add("J2EE");
System.err.println("unsorted ArrayList in Java : " + unsortedList);
//Sorting ArrayList in ascending Order in Java
Collections.sort(unsortedList);
System.out.println("Sorted ArrayList in Java - Ascending order : " + unsortedList);
//Sorting ArrayList in descending order in Java
Collections.sort(unsortedList, Collections.reverseOrder());
System.err.println("Sorted ArrayList in Java - Descending order : " + unsortedList);
}
}
Output:
unsorted ArrayList in Java : [Java, C++, J2EE]
Sorted ArrayList in Java - Ascending order : [C++, J2EE, Java]
Sorted ArrayList in Java - Descending order : [Java, J2EE, C++]
That's all on How to Sort ArrayList in Java on both ascending and descending order. Just remember that Collections.sort() will sort the ArrayList in ascending order and if you provide reverse comparator it will sort the ArrayList in descending order in Java.
5) What is double checked locking in Singleton? Answer : Interviewer will never stop asking this question. It's mother of all frequently asked question in Java. Singleton means we can create only one instance of that class,in term of singleton DCL is the way to ensure that at any cost only one instance is created in multi-threaded environment its possible that simultaneously two thread trying to create instance of singleton class in that situation we cant sure that only one instance is created so avoid this situation using double checked locking by using synchronized block where we creating the object.
Code Example :
class SingletonClass { private DCL dcl = null; public DCL getDCL() { if (dcl == null) { synchronized { if (dcl == null) dcl = new DCL(); } } return dcl; } }
To learn more about why double checked locking was broken before Java 1.5, see this article.
6) How do you create thread-safe Singleton in Java? Answer : There are more than one ways to do it. You can create thread safe Singleton class in Java by creating the one and only instance during class loading. static fields are initialized during class loading and Classloader will guarantee that instance will not be visible until its fully created.
7) When to use volatile variable in Java? Answer : Volatile keyword is used with only variable in Java and it guarantees that value of volatile variable will always be read from main memory and not from Thread's local cache. So we can use volatile to achieve synchronization because its guaranteed that all reader thread will see updated value of volatile variable once write operation completed, without volatile keyword different reader thread may see different values. Volatile modifier also helps to prevent reordering of code by compiler and offer visibility guarantee by happens-before relationship. See this article to learn more about volatile in Java.
8) When to use transient variable in Java? Answer : Transient in Java is used to indicate that the variable should not be serialized. Serialization is a process of saving an object's state in Java. When we want to persist and object's state by default all instance variables in the object is stored. In some cases, if you want to avoid persisting some variables because we don’t have the necessity to transfer across the network. So, declare those variables as transient. If the variable is declared as transient, then it will not be persisted. This is the main purpose of the transient keyword, to learn more about transient variable in Java, see this tutorial.
9) Difference between transient and volatile variable in Java? Answer :
Transient variable : transient keyword is used with those instance variable which will not participate in serialization process.we cannot use static with transient variable as they are part of instance variable.
Volatile variable : volatile keyword is used with only variable in Java and it guarantees that value of volatile variable will always be read from main memory and not from Thread's local cache, it can be static. to learn more differences and answer this question in detail, see here.
10) Difference between Serializable and Externalizable in Java? Answer :
Serialization is a default process of serializing or persisting any object's state in Java.
It's triggered by implementing Serializable interface which is a marker interface (an interface without any method).
While Externalizable is used to customize and control default serialization process which is implemented by application.
Main difference between these two is that Externalizable interface provides complete control to the class implementing the interface whereas Serializable interface normally uses default implementation to handle the object serialization process.
Externalizable interface has two method writeExternal(ObjectOutput) and readExternal(ObjectInput) method which are used to handle customized object serialize process and in terms of performance its good because everything is under control. to learn more about this classical question, see this answer as well.
11) Can we override private method in Java? Answer : No, we cannot override private methods in Java as if we declare any variable ,method as private that variable or method will be visible for that class only and also if we declare any method as private than they are bonded with class at compile time not in run time so we cant reference those method using any object so we cannot override private method in Java.
12) Difference between Hashtable and HashMap in Java? Answer : This is another frequently asked question from Java interview. Main difference between HaspMap and Hashtable are following :
- HashMap allows null values as key and value whereas Hashtable doesn't allow nulls.
- Hashtable is thread-safe and can be shared between multiple threads whereas HashMap cannot be shared between multiple threads without proper synchronization.
- Because of synchronization, Hashtable is considerably slower than HashMap, even in case of single threaded application.
- Hashtable is a legacy class, which was previously implemented Dictionary interface. It was later retrofitted into Collection framework by implementing Map interface. On the other hand, HashMap was part of framework from it's inception.
- You can also make your HashMap thread-safe by using Collections.synchronizedMap() method. It's performance is similar to Hashtable.
See here to learn more and understand when to use Hashtable and HashMap in Java.
13) Difference between List and Set in Java? Answer : List and set both are very useful interfaces of collections in Java and difference between these two is list allows duplicate element but set don't allows duplicate elements another difference is list maintain the insertion order of element but set is unordered collection .list can have many null objects but set permit only one null element. This question is some time also asked as difference between Map, List and Set to make it more comprehensive as those three are major data structure from Java's Collection framework. To answer that question see this article.
14) Difference between ArrayList and Vector in Java Answer : Vector and ArrayList both implement the list interface but main difference between these two is vector is synchronized and thread safe but list is not because of this list is faster than vector.
15) Difference between Hashtable and ConcurrentHashMap in Java? Answer : Both Hashtable and ConcurrentHashMap is used in multi-threaded environment because both are therad-safe but main difference is on performance Hashtable's performance become poor if the size of Hashtable become large because it will be locked for long time during iteration but in case of concurrent HaspMap only specific part is locked because concurrent HaspMap works on segmentation and other thread can access the element without iteration to complete. To learn more about how ConcurrentHashMap achieves it's thread-safety, scalability using lock stripping and non blocking algorithm, see this article as well.
16) Which two methods you will override for an Object to be used as Key in HashMap? Answer : equals() and hashCode() methods needs to be override for an object to be used as key in HaspMap. In Map objects are stored as key and value. put(key ,value) method is used to store objects in HashMap at this time hashCode() method is used to calculate the hash-code of key object and both key and value object is stored as map.entry.if two key objects have same hash-code then only value object is stored in that same bucket location but as a linked list value is stored and if hash code is different then another bucket location is created. While retrieving get(key) method is used at this time hash code of key object is calculated and then equals() method is called to compare value object. to learn more about how get() method of HashMap or Hashtable works, see that article.
17) Difference between wait and sleep in Java? Answer: Here are some important differences between wait and sleep in Java
- wait() method release the lock when thread is waiting but sleep() method hold the lock when thread is waiting.
- wait() is a instance method and sleep is a static method .
- wait method is always called from synchronized block or method but for sleep there is no such requirement.
- waiting thread can be awake by calling notify() and notifyAll() while sleeping thread can not be awaken by calling notify method.
- wait method is condition based while sleep() method doesn't require any condition. It is just used to put current thread on sleep.
- wait() is defined in java.lang.Object class while sleep() is defined in java.lang.Thread class
18) Difference between notify and notifyAll in Java? Answer : main difference between notify and notifyAll is notify method will wake up or notify only one thread and notifyall will notify all threads. If you are sure that more than one thread is waiting on monitor and you want all of them to give equal chance to compete for CPU, use notifyAll method. See here more differences between notify vs notifyAll.
19) What is load factor of HashMap means? Answer : HashMap's performance depends on two things first initial capacity and second load factor whenever we create HashMap initial capacity number of bucket is created initially and load factor is criteria to decide when we have to increase the size of HashMap when its about to get full.
20) Difference between PATH and Classpath in Java?
Answer : PATH is a environment variable in Java which is used to help Java program to compile and run.To set the PATH variable we have to include JDK_HOME/bin directory in PATH environment variable and also we cannot override this variable.
On the other hand, ClassPath variable is used by class loader to locate and load compiled Java codes stored in .class file. We can set classpath we need to include all those directory where we have put either our .class file or JAR file which is required by your Java application,also we can override this environment variable.
21) Difference between extends Thread and implements Runnable in JavaYou will see this question as first or second on multi-threading topic. One of the main point to put across while answering this question is Java's multiple inheritance support. You cannot more than one class, but you can implement more than one interface. If you extend Thread class just to override run() method, you lose power of extending another class, while in case of Runnable, you can still implement another interface or another class. One more difference is that Thread is abstraction of independent path of execution, while Runnable is abstraction of independent task, which can be executed by any thread. That's why it's better to implement Runnable than extending Thread class in Java. If you like to dig more, see this answer.
Hashtable doesn't permit any sort of nulls (key or values).